Who doesn’t like a good old fashioned Robot or Droid?
It’s about time I pulled my finger out and made some.
K2SO – Bender – Pit Droid – Tinny Tim – Back to Top
For my Droids, I am currently using a company called Droid Division (DD) for my Droid files. Once the files have been purchased you can decide on build scale and material that you want to print. I have to say DD .stl files are great to work with and all the parts fit together extremely well, so no headaches. For starters, I decided on their Security Droid.. i.e. K2SO from Star Wars – Rouge One, A full size K2SO is available to print, but I have just opted for the head for now as a full size K2SO is about 7′ high and currently I just don’t have the time to print something that big, so for now just the head it is.
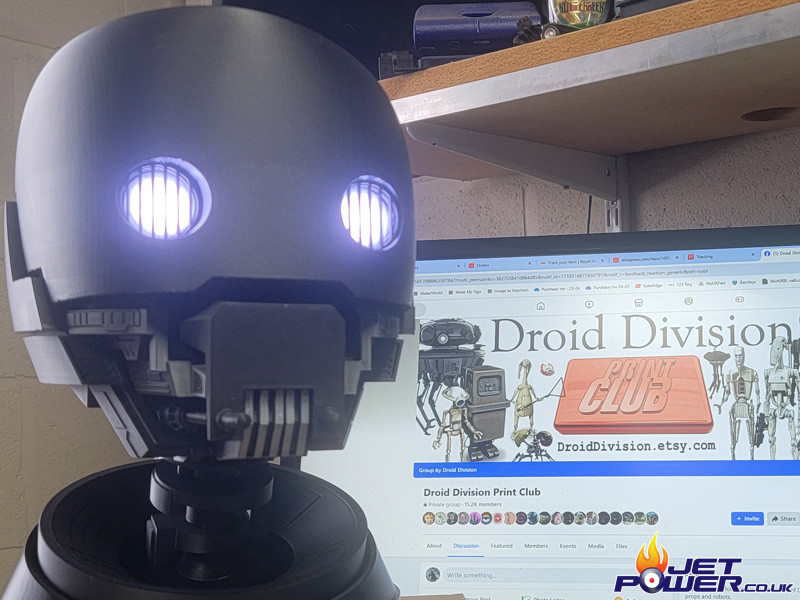
There is an option for those folk that are inclined to print a Pan/ Tilt mechanism for some illuminated eyes, very subtle, but looks awesome and makes the Droid come alive. Two mini servos are used for this and can be driven by an Arudino, the eyes lights are circular 7 LED, Neo Pixels or Jewels which are RGB units and the colour can be set by software.
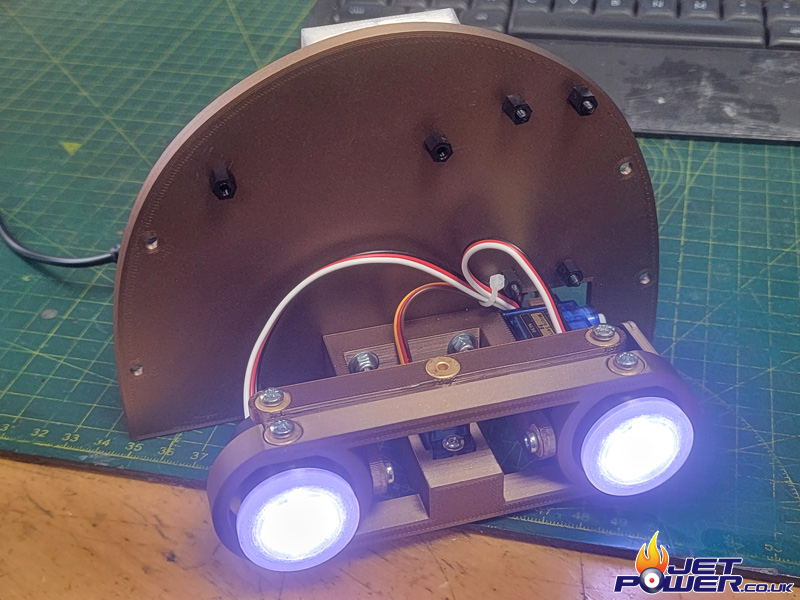
To randomly animate the eyes I made use of a ESP32 micro controller, similar to an Arduino and in fact can use the Arduino IDE software for programming. The code for this can be found in one of the DD Security Droid folders.
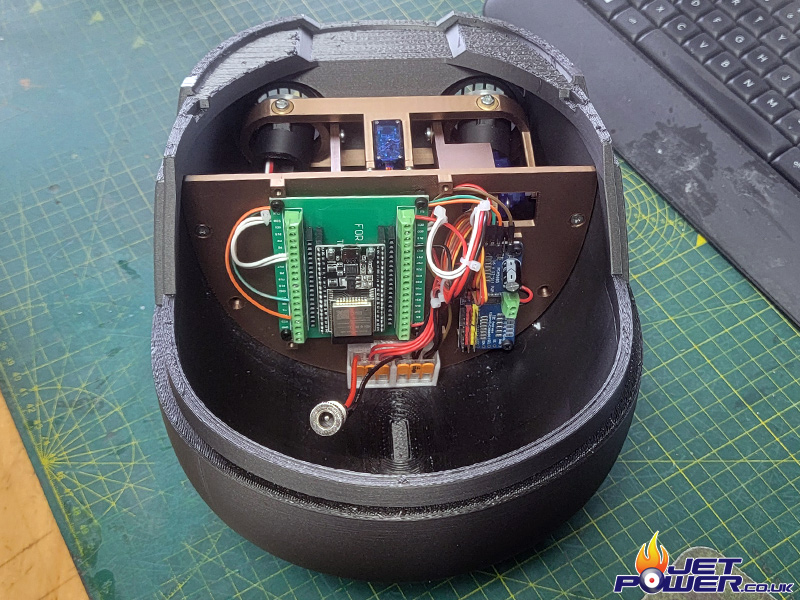
Inside view of the Pan and Tilt Mechanism to the left and the ESP32 mounted to the right.
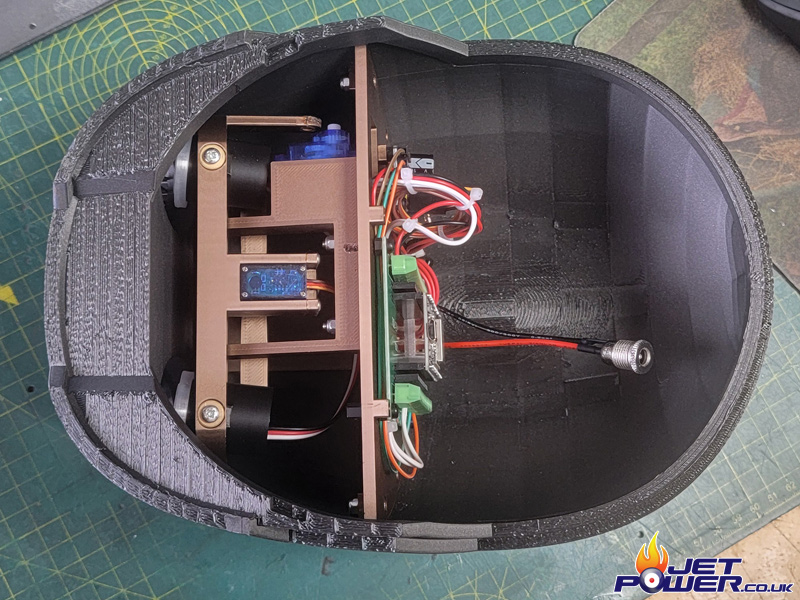
K2SO – Bender – Pit Droid – Tinny Tim – Back to Top
Who doesn’t like Futurama?!
One of my favorite characters is Bender Rodriguez, or DD Bender Robot.
I printed him at 75% scale, for filament usage, time and effort this was about right for me and I think he came out great. Some of the more accomplished DD builders will take the time to sand and paint these Droids, but unless it’s for a Film or TV, that’s not for me. I have 3 jobs I want to do with him, animate the eyes, get motion in to the head and build his chest in to a peltier module fridge cooler.
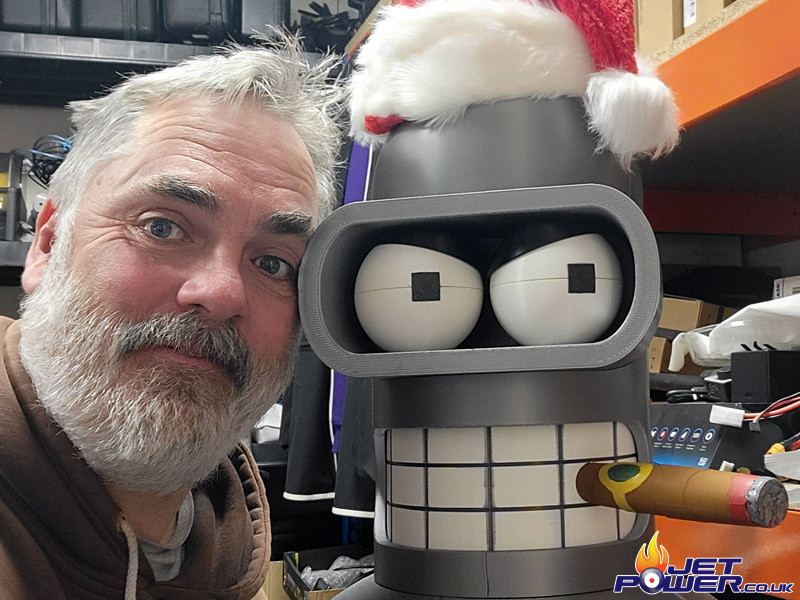
You just can’t beat a Festive Bender !
Sounds so wrong, which Bender would of course approve.
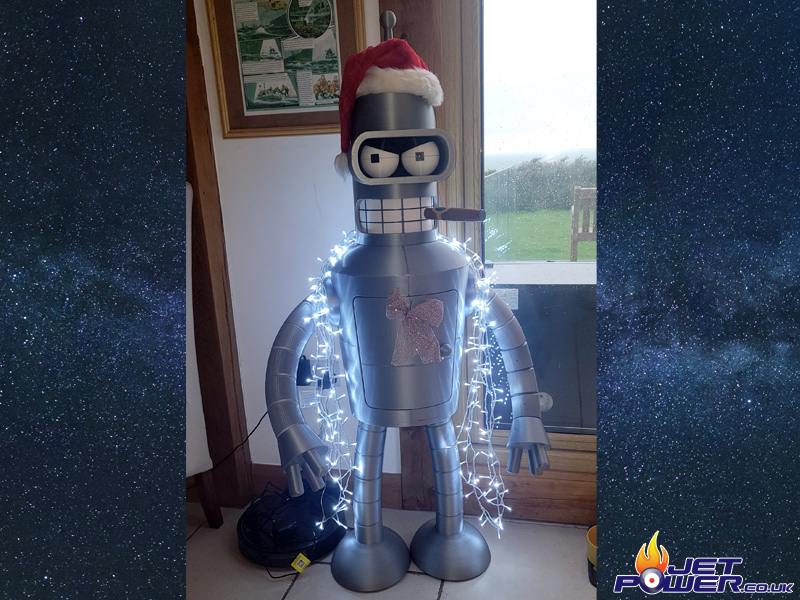
I think Bender needs more alcohol (Fuel)
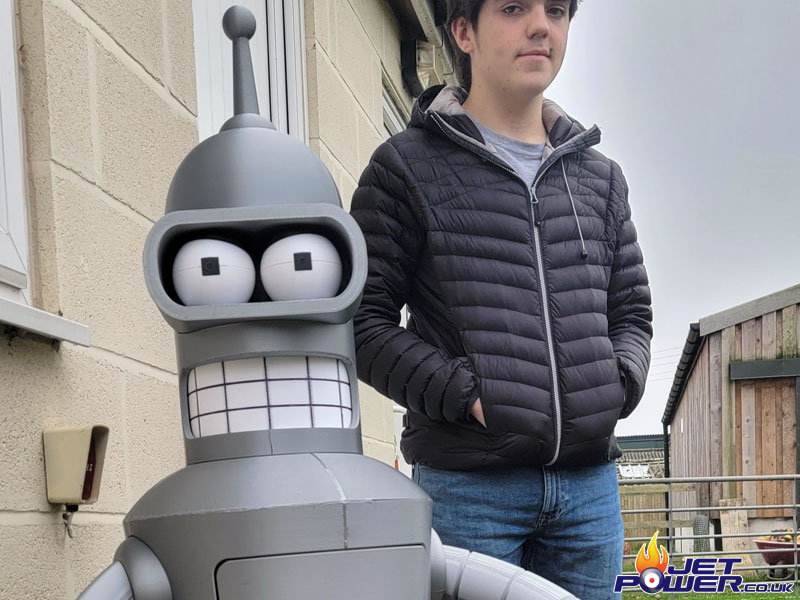
K2SO – Bender – Pit Droid – Tinny Tim – Back to Top
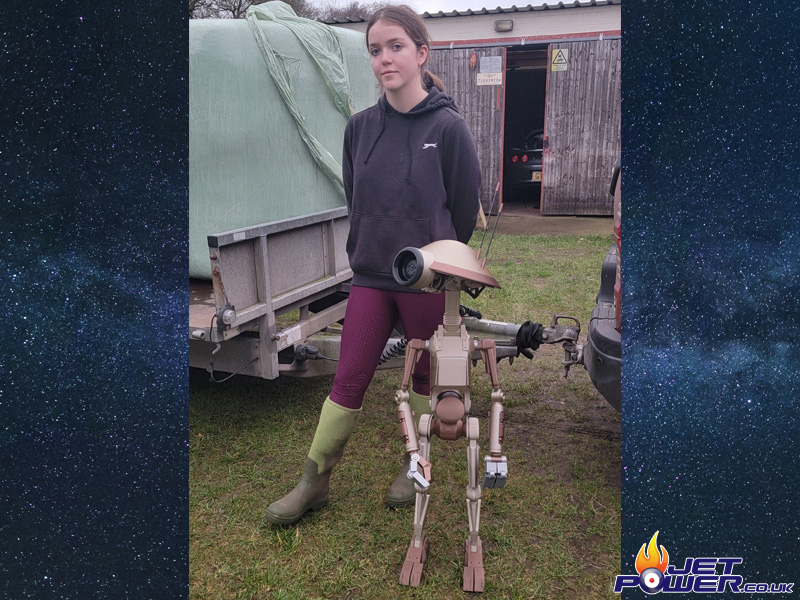
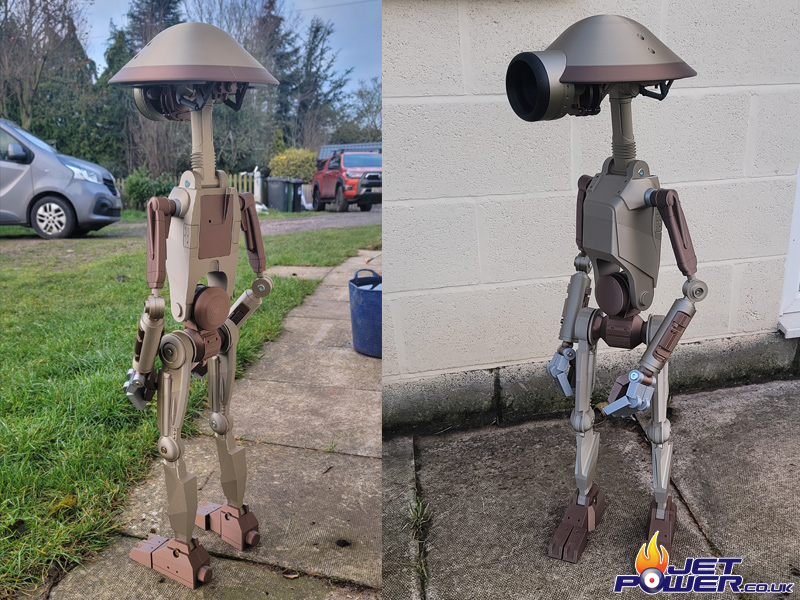
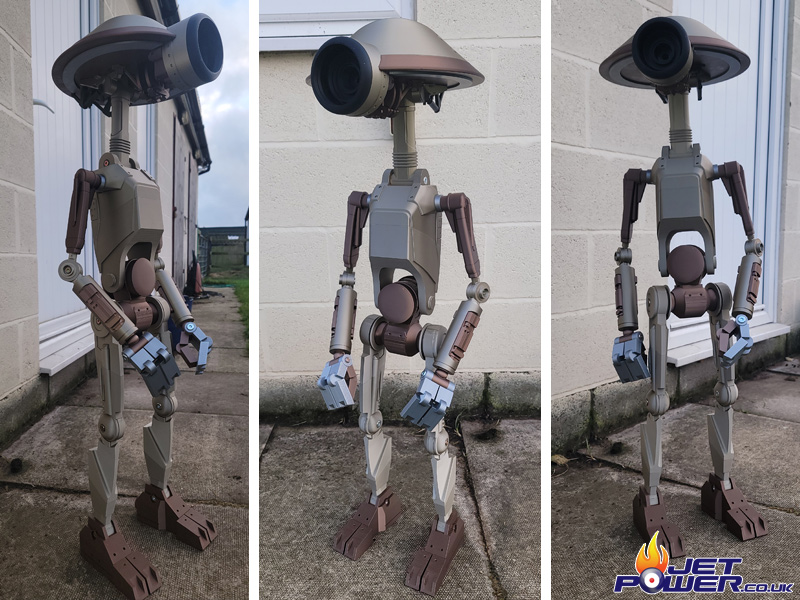
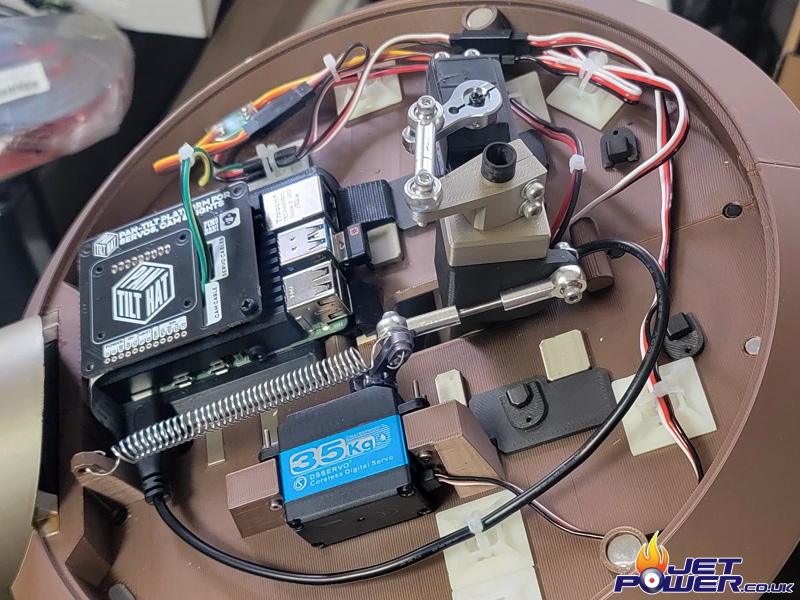
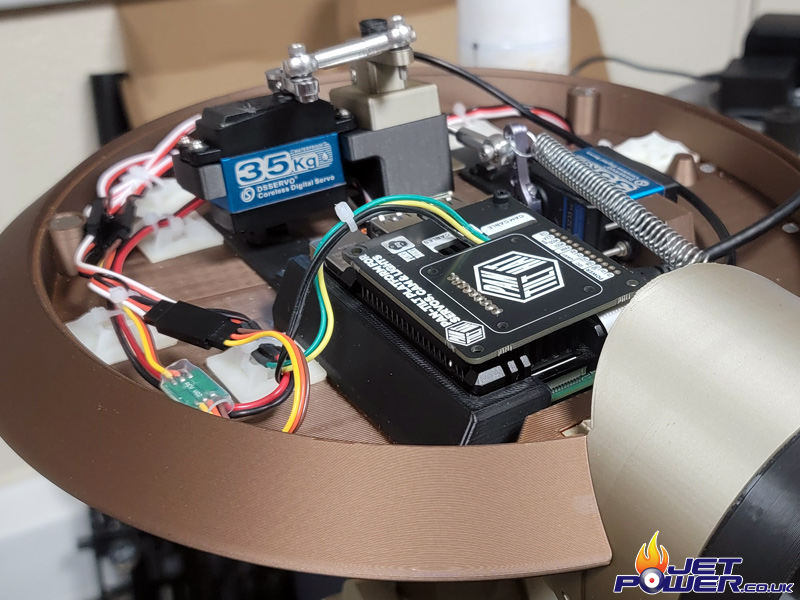
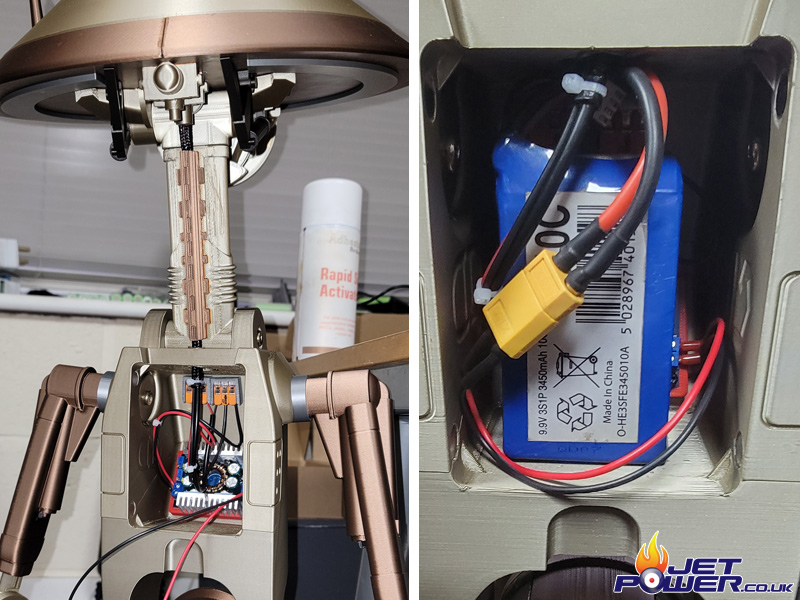
K2SO – Bender – Pit Droid – Tinny Tim – Back to Top
Another Futurama Droid, “Tinny Tim Bot”. An easy build from DD and I built him at 100% scale. Again, no painting from me and just metallic filament, I think I have done well with the colour choice and I am very happy with the outcome, he just needs some animated eyes which will follow.
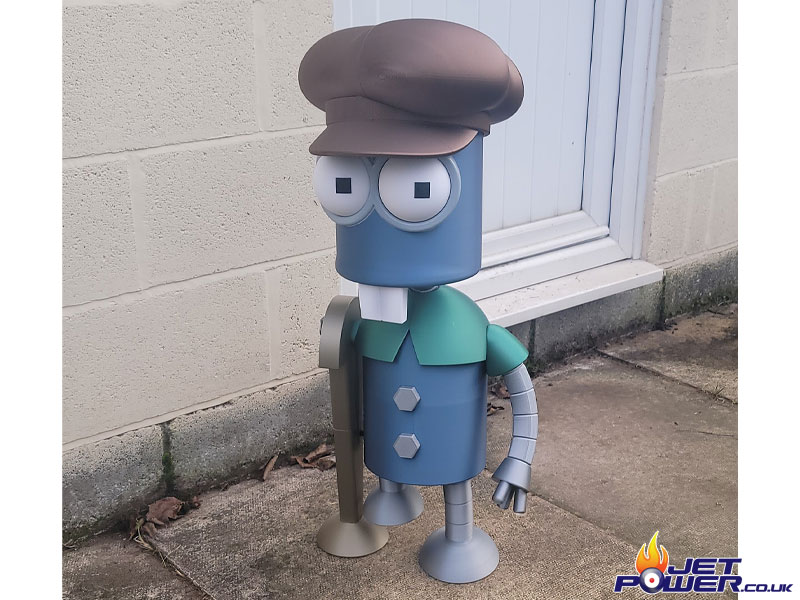
# Oh, crumb.
# You raised my hopes and dashed them quite expertly, sir – Bravo
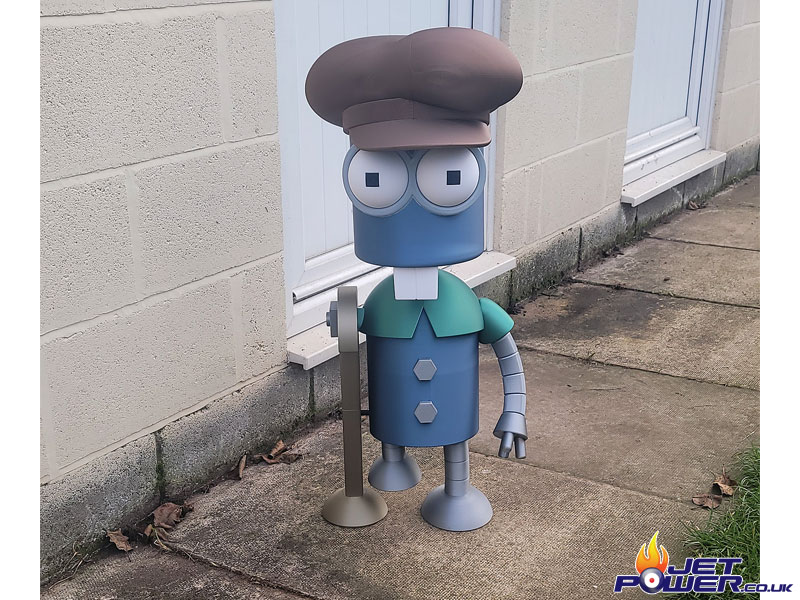